반응형
https://www.hackerrank.com/challenges/grading/problem
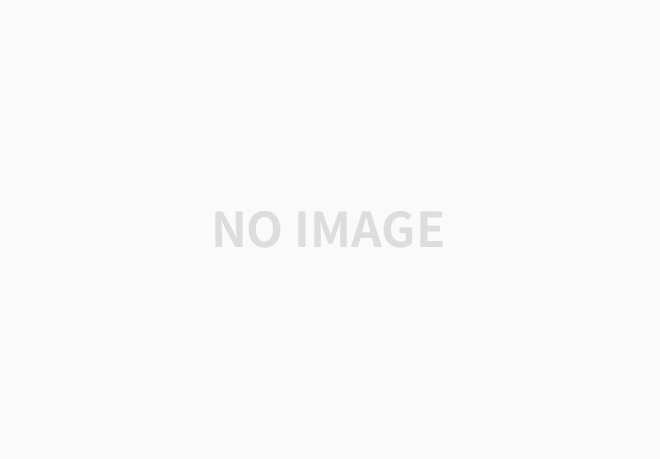
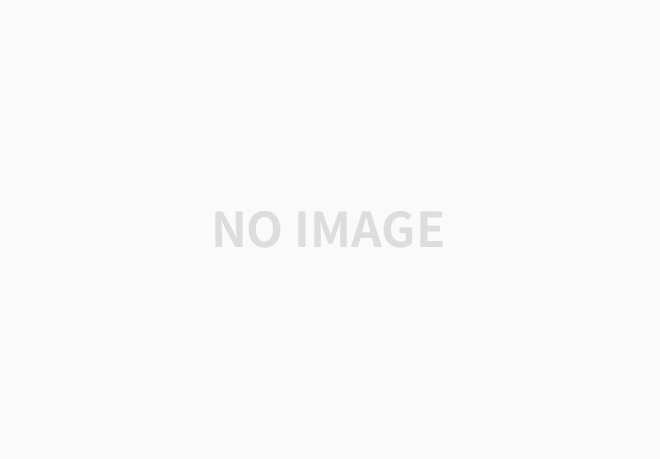
#include <bits/stdc++.h> using namespace std; string ltrim(const string &); string rtrim(const string &); /* * Complete the 'gradingStudents' function below. * * The function is expected to return an INTEGER_ARRAY. * The function accepts INTEGER_ARRAY grades as parameter. */ vector<int> gradingStudents(vector<int> grades) { int sNum = grades.size(); vector<int> roundedGrade(sNum); for (int i = 0; i < sNum; i++) { if (grades[i] < 38) { roundedGrade[i] = grades[i]; } else { int re = grades[i] % 5; if (re > 2) { // when re == 4 or re == 3 roundedGrade[i] = grades[i] + (5 - re); } else { roundedGrade[i] = grades[i]; } } } return roundedGrade; } int main() { ofstream fout(getenv("OUTPUT_PATH")); string grades_count_temp; getline(cin, grades_count_temp); int grades_count = stoi(ltrim(rtrim(grades_count_temp))); vector<int> grades(grades_count); for (int i = 0; i < grades_count; i++) { string grades_item_temp; getline(cin, grades_item_temp); int grades_item = stoi(ltrim(rtrim(grades_item_temp))); grades[i] = grades_item; } vector<int> result = gradingStudents(grades); for (size_t i = 0; i < result.size(); i++) { fout << result[i]; if (i != result.size() - 1) { fout << "\n"; } } fout << "\n"; fout.close(); return 0; } string ltrim(const string &str) { string s(str); s.erase( s.begin(), find_if(s.begin(), s.end(), not1(ptr_fun<int, int>(isspace))) ); return s; } string rtrim(const string &str) { string s(str); s.erase( find_if(s.rbegin(), s.rend(), not1(ptr_fun<int, int>(isspace))).base(), s.end() ); return s; }
반응형
'Devlog > Coding Practice' 카테고리의 다른 글
[C++][Implementation] Number Line Jumps (0) | 2021.07.30 |
---|---|
[C++][Implementation] Apple and Orange (0) | 2021.07.28 |
[C++] 1193 분수찾기 (0) | 2021.03.09 |
[C++][Warmup] Time Conversion (0) | 2020.09.06 |
[C++] 10996 별 찍기 - 21 (0) | 2020.08.10 |